Next.js has experimental support for Edge API Routes which means your API routes can run at the Edge with greater performance (low latency, no cold starts), backed by standard Web APIs.
One benefit to Edge computing is the reduced latency. No longer do you need to make trips around the world to a data center but now compute requests closest to you, the user. Another is the lack of cold starts with Grafbase which means your API responses are blazingly fast!
The Grafbase GraphQL API runs at the Edge which means your database, and frontend can communicate with reduced latency that improves user experience.
In this guide we'll create a Next.js Edge API route that talks to your serverless GraphQL API deployed to Grafbase.
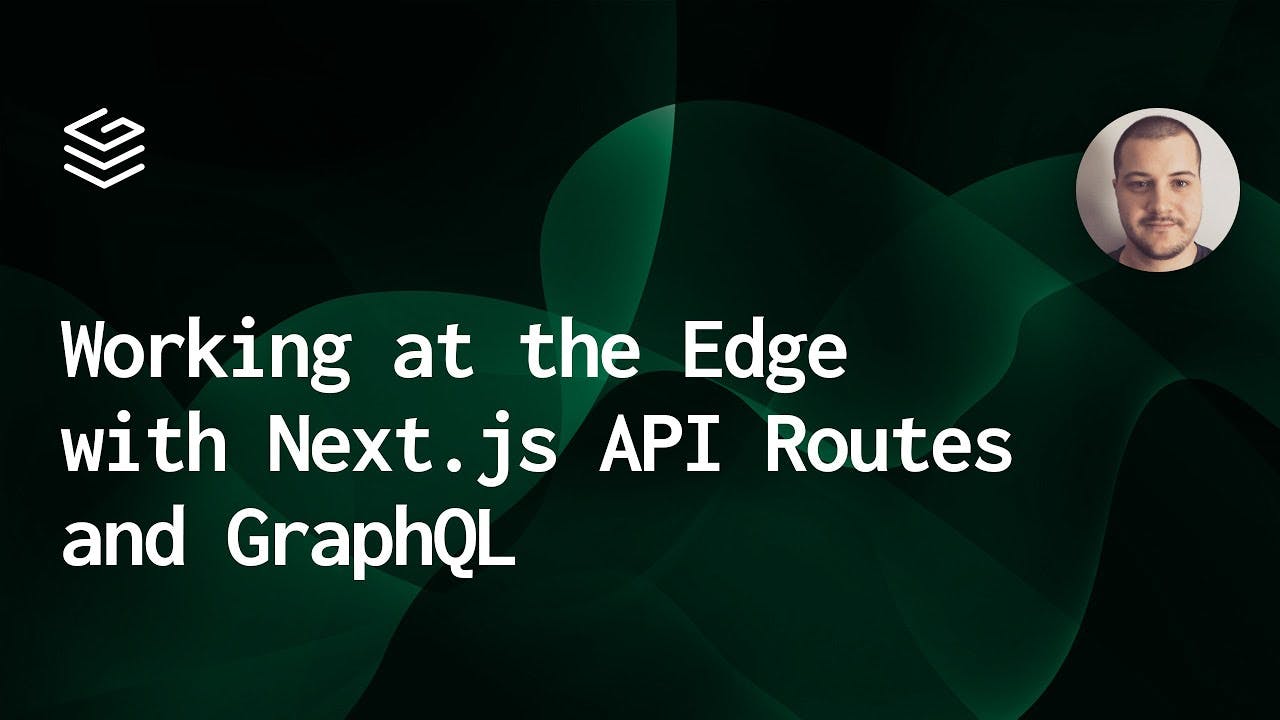
Let's get started by creating a new TypeScript Next.js project:
npx create-next-app@latest --typescript nextjs-edge-api-routes
cd nextjs-edge-api-routes
Now create the file post.ts
inside pages/api
:
touch pages/api/post.ts
We'll be using the Blog template schema deployed to Grafbase.
You'll need to set the GRAFBASE_API_URL
and GRAFBASE_API_KEY
environment variables inside of the .env
file in the root of your project. You can get these from your project settings.
GRAFBASE_API_URL=
GRAFBASE_API_KEY=
Next using the GraphQL API deployed to the Edge with Grafbase, we can execute the following query to get posts and comments:
query GetAllPosts($first: Int!) {
postCollection(first: $first) {
edges {
node {
title
comments(first: 10) {
edges {
node {
content
}
}
}
}
}
}
}
Notice that we're using variables with our query.
Add this to posts.ts
as the const query
:
const query = `
query GetAllPosts($first: Int!) {
postCollection(first: $first) {
edges {
node {
title
comments(first: 10) {
edges {
node {
content
}
}
}
}
}
}
}
`
Next we'll add to the same file the handler
function that:
- Makes a
fetch
request to your Grafbase API URL - Uses the
POST
HTTP method - Passes the
query
andvariables
in the body - Passes the header
x-api-key
with your Grafbase API Key - Returns the
fetch
request inside of the handler
export default async function handler() {
return fetch(process.env.GRAFBASE_API_URL, {
method: 'POST',
body: JSON.stringify({
query,
variables: { first: 10 },
}),
headers: {
'x-api-key': process.env.GRAFBASE_API_KEY,
},
})
}
To make this work at the Edge, we'll set the runtime to experimental-edge
inside of the exported config
object:
export const config = {
runtime: 'edge',
}
Once deployed to Vercel, the API route will talk to Grafbase and return all posts at the Edge!