Grafbase Resolvers are a powerful way to build your own GraphQL API. We can use resolvers to compute custom business logic, make network requests, invoke dependencies, and more.
Turso is a distributed database that is hosted on the edge, built on libSQL (an open contribution fork of SQLite).
Its primary aim is to diminish the delay in query responses in applications that handle requests from worldwide locations. Given that Grafbase situates your project's API at the edge through its edge gateway, it's logical to pair Turso with Grafbase Resolvers. This combination allows for optimal storage and retrieval of data in close proximity to your users.
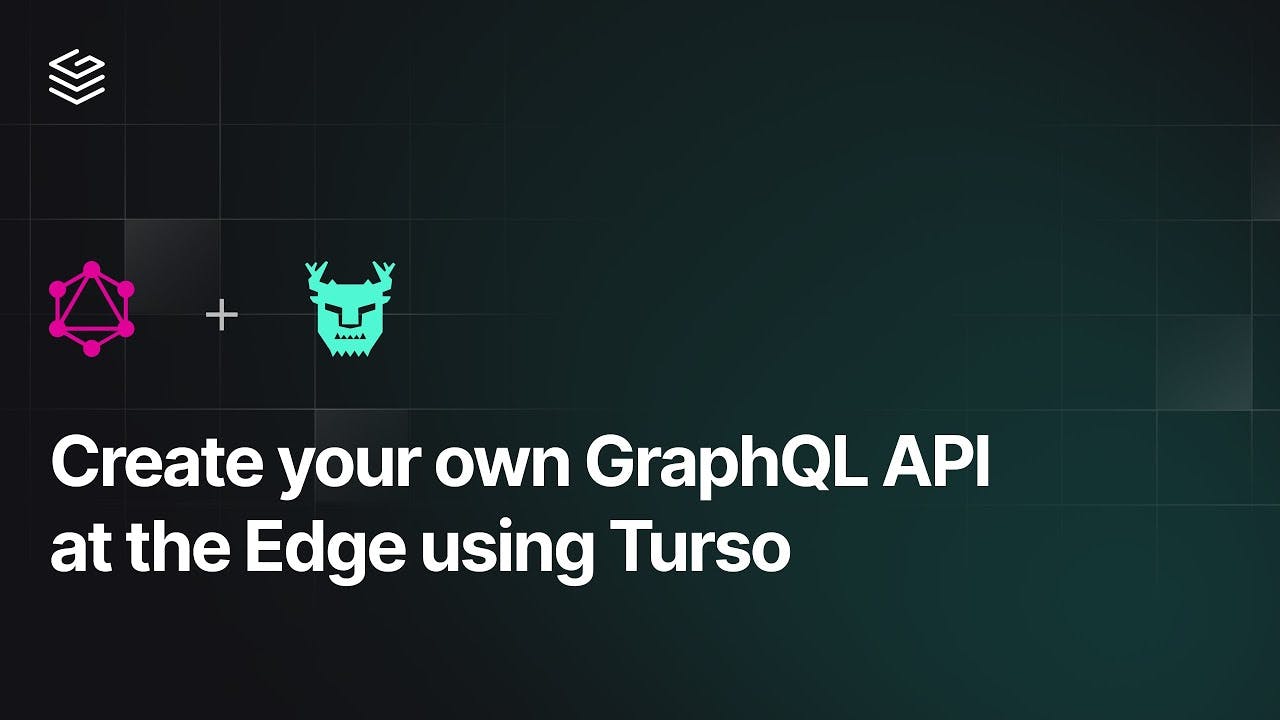
In this guide, we'll explore using the @libsql/client
library inside Grafbase Edge Resolvers.
Turso make it really easy to create a database. Begin by installing the CLI:
brew install chiselstrike/tap/turso
Next login. You'll be redirected to Turso's website to login:
turso auth login
Once authenticated, you are ready to create your first database:
turso db create my-db
Turso will select a default location for the database based on your physical location.
We'll connect to the database shell using the Turso CLI to create a new users
table:
turso db shell my-db
Now type the following SQL statement into the shell:
create table users (name text);
We won't add any data using the shell but instead do that using GraphQL.
If you don't already have a Grafbase project created, run the following inside of a new or existing directory:
npx grafbase init
Inside grafbase/schema.graphql
replace the contents with the following GraphQL user
type.
This type represents our Turso users
table we created earlier:
type User {
name: String
}
Finally, create the file grafbase/.env
. Add the LIBSQL_DB_URL
and LIBSQL_DB_AUTH_TOKEN
environment variables.
LIBSQL_DB_URL=libsql://...
LIBSQL_DB_AUTH_TOKEN=...
You can get these values using the Turso CLI:
turso db show my-db --url
turso db tokens create my-db -e none
Inside the grafbase
directory you will want to install the @libsql/client
dependency.
npm init -y
npm install -E @libsql/client
The @libsql/client
library allows you to query a Turso database.
We'll begin by creating defining a GraphQL schema that will match what we have in our Turso database.
In the future we will explore introspecting the database automatically but hopefully this shows how you can have greater control over how your backend looks.
Inside grafbase/schema.graphql
add the following:
extend type Mutation {
createUser(name: String!): User! @resolver(name: "create")
}
Now create the file grafbase/resolvers/create.ts
and add the following:
import { createClient } from '@libsql/client/web'
const client = createClient({
url: process.env.LIBSQL_DB_URL,
authToken: process.env.LIBSQL_DB_AUTH_TOKEN,
})
export default async function CreateUserResolver(_, { name }) {
await client.execute({
sql: 'insert into users values (?)',
args: [name],
})
return { name }
}
We now have everything we need to make a request. Make sure to run the Grafbase development server using npx grafbase dev
and run the following request within Pathfinder:
mutation {
createUser(name: "Jamie") {
name
}
}
Now we've some data in our Turso database, we'll move onto creating a GraphQL query to fetch the data.
Inside grafbase/schema.graphql
add the following:
extend type Query {
users: [User!] @resolver(name: "users")
}
Now create the file grafbase/resolvers/users.ts
and add the following:
import { createClient } from '@libsql/client/web'
const client = createClient({
url: process.env.LIBSQL_DB_URL,
authToken: process.env.LIBSQL_DB_AUTH_TOKEN,
})
export default async function UsersResolver() {
try {
const { rows } = await client.execute('select * from users')
return rows
} catch (err) {
return []
}
}
Now you can run the following query to fetch all users from your database:
{
users {
name
}
}
That's it! You're now ready to begin integrating databases like Turso into your own Grafbase API, taking advantage of edge caching and more.