The GraphQL ecosystem continues to grow exponentially, introducing an ever-expanding array of developer tools and services. To help you get the most out of building with GraphQL, we've curated a short list of libraries and services you should be using in 2023.
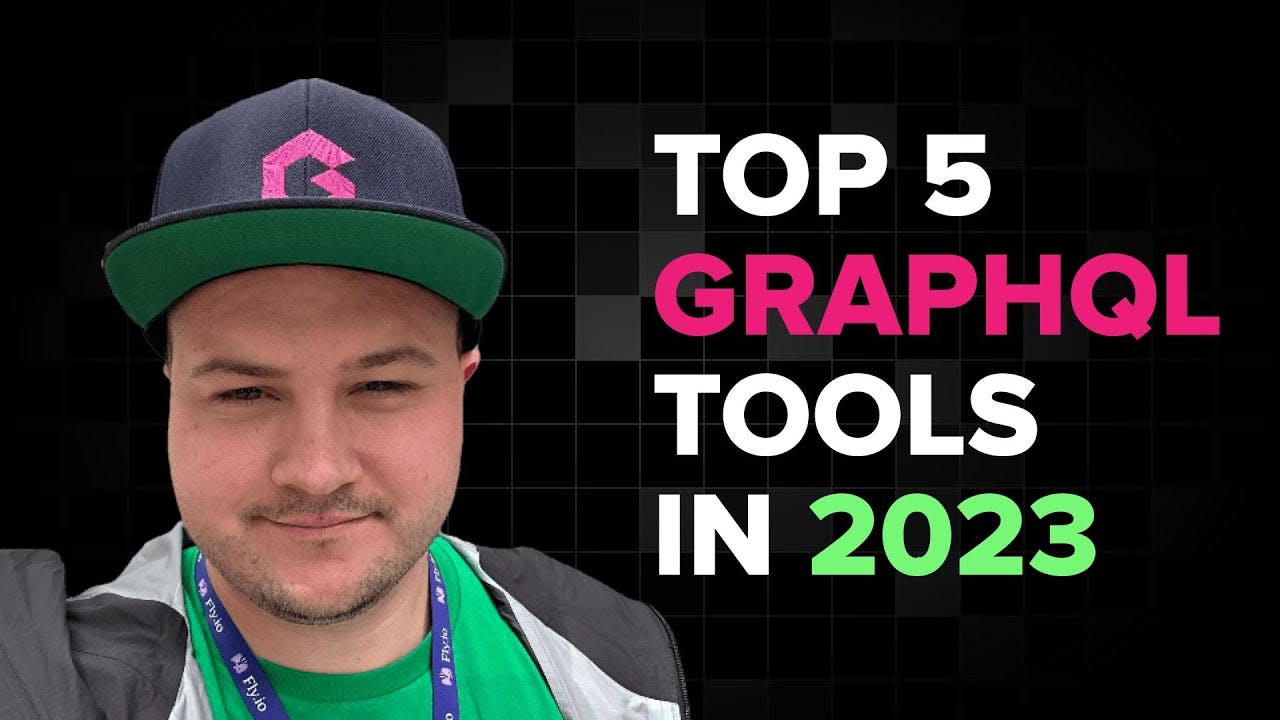
Unlock the potential of modern web and mobile app development by seamlessly combining REST and GraphQL APIs. With Grafbase, effortlessly unify API designs and expose a single GraphQL API, empowering your application development process.
OpenAPI, previously Swagger, is an open standard that simplifies API definition and documentation by describing APIs in a machine-readable format, facilitating developer understanding and interaction with diverse APIs. Meanwhile, Grafbase Edge Gateway seamlessly merges OpenAPI-driven REST APIs and converts them to GraphQL, amplifying the capabilities of your stack.
extend schema
@openapi(
name: "Stripe"
schema: "https://raw.githubusercontent.com/stripe/openapi/master/openapi/spec3.json"
headers: [
{ name: "Authorization", value: "Bearer {{ env.STRIPE_API_KEY }}" }
]
) {
query: Query
}
It's free to get started. Try it now with the Stripe OpenAPI spec and Grafbase CLI:
npx grafbase init --template openapi-stripe
Learn more about the OpenAPI Connector.
The built-in type system of GraphQL is one of its most significant advantages. It simplifies the process of providing TypeScript types for our entire GraphQL schema and individual operations within our application. Previously, we relied on the GraphQL Code Generator to generate hooks for existing GraphQL clients, but this approach made it challenging to adopt new GraphQL clients.
Now, the GraphQL Code Generator recommends using the client-preset
along with TypedDocumentNode
to enhance functions and enable the inference of correct operation and variable types.
This approach also encourages keeping operations and fragments within their respective components. By following this methodology, we can reduce code complexity and technical debt, establish stricter component type dependencies, and effortlessly migrate to any GraphQL client that supports TypedDocumentNode
.
export const LessonFragment = graphql(/* GraphQL */ `
fragment LessonItem on Lesson {
title
}
`)
export function Lesson(props: { lesson: FragmentType<typeof LessonFragment> }) {
const lesson = useFragment(LessonFragment, props.lesson)
return (
// Fully typed!
<li>{lesson.title}</li>
)
}
It's completely open source, check it out.
If writing GraphQL operations and configuring codegen for TypeScript is slowing you down, consider replacing your GraphQL client with no GraphQL client and use GQty.
GQty will automatically transform the below into a GraphQL operation under the hood.
import type { FunctionComponent } from 'react'
import { useQuery } from '../gqty/'
const Query: FunctionComponent = () => {
const {
me: { name, friends },
} = useQuery()
return (
<>
Hello {name}!
<ol>
{friends().map(user => (
<li key={user.id}>{user.name}</li>
))}
</ol>
</>
)
}
export default Query
GQty is also open source, check it out.
For a long time, developers have grappled with distinguishing /graphql
requests in the Devtools Network Inspector. Fortunately, those challenging days are behind us.
The GraphQL Network Inspector, an exceptional extension for developer tools, brings to light the categories of requests (queries, mutations, and subscriptions) and employs the GraphQL operation name, simplifying the debugging process.
The GraphQL Network Inspector simplifies the debugging process while developing GraphQL web and mobile applications on the client. It provides valuable information about the request, variables, search payloads, filter responses, and more, offering insights that aid in troubleshooting and improving the application.
It's completely open source, check it out.
Many GraphQL developers utilize various APIs while working in teams, collectively creating and sharing GraphQL operations. Collaborative tools like Insomnia and Postman simplify this process, yet crafting queries and mutations for a GraphQL API can still be time-consuming and unexciting.
To address this challenge, our friends at Escape developed GraphMan, an innovative solution that eliminates the burdensome aspects. With GraphMan, you can effortlessly generate a comprehensive collection from a single GraphQL endpoint. Each collection includes a request for every query and mutation, conveniently pre-populated with fields, parameters, and variables.
GraphMan uses the Deno runtime. Create a collection passing your Grafbase API URL to the command below:
deno run https://deno.land/x/graphman@v1.2.1/src/cli.ts GRAFBASE_API_URL_HERE
GraphMan is free to use and is completely open source, check it out.